Note
Click here to download the full example code
Example: Night Train from Amsterdam to Zürich¶
Standard schedule 2021/22 from Amsterdam over Emmerich, Basel to Zürich (a single route) on fridays, saturdays and sundays:
Route : XNAC-EEM-RXBA-XSZH
Calendar : 12/12/2021 to 12/12/2022 Fri, Sat, Sun
Start at: 20:00 in XNAC
Arrival at: 21:30 in EEM
Arrival at: 03:30 in RXBA
Arrival at: 04:30 in XSZH
Update schedule due to construction work between Amsterdam and Utrecht on sundays from 12/04/2022 on:
The train on sundays starts in Utrecht with destination Basel and handover Venlo. Therefore we need to routes from April on:
Route : XNAC-EEM-RXBA-XSZH
Calendar : 12/04/2022 to 12/12/2022 Fri, Sat
Start at: 20:00 in XNAC
Arrival at: 21:30 in EEM
Arrival at: 03:30 in RXBA
Arrival at: 04:30 in XSZH
Route : XNU-XNVL-RXBA
Calendar : 12/04/2022 to 12/12/2022 Sun
Start at: 20:30 in XNU
Arrival at: 22:00 in XNVL
Arrival at: 04:00 in RXBA
from tom.plot import plot_train, plot_graph
from tom.tom import make_train_from_yml, TrainRun, RouteSection, Route
from tom.util import example, dump_routing_info_as_xml
Standard schedule (version 1)¶
Load example night train 2021/22 standard schedule (version 1) from yaml specification
_, t_spec_file = example('../tests/data', 'ac-zue-1')
print(t_spec_file.read_text())
Out:
---
# Nighttrain from Amsterdam to Zürich
coreID: 402403
version: 1
sections:
- departure_station: XNAC
arrival_station: EEM
id: 10
version: 1
departure_time: '20:00:00'
travel_time: '01:30:00'
calendar:
begin: &b-begin '2021-12-12'
end: &b-end '2021-12-31'
mask: 'Fri Sat Sun'
color: green
succ:
- 11
- departure_station: EEM
arrival_station: RXBA # Basel Grenze
id: 11
version: 1
travel_time: '06:00:00'
succ:
- 12
- departure_station: RXBA
arrival_station: XSZH # Zürich HB
id: 12
version: 1
travel_time: '01:00:00'
Create train object and show its train id
t = make_train_from_yml(t_spec_file)
t.train_id()
Out:
'TR/8350/402403/00/2021'
Bildfahrplan¶
Show timetable as plot. No sections from Utrecht over Venlo here.
stations = ['XNAC', 'XNU', 'EEM', 'XNVL', 'RXBA', 'XSZH']
plot_train(t, all_stations=stations)
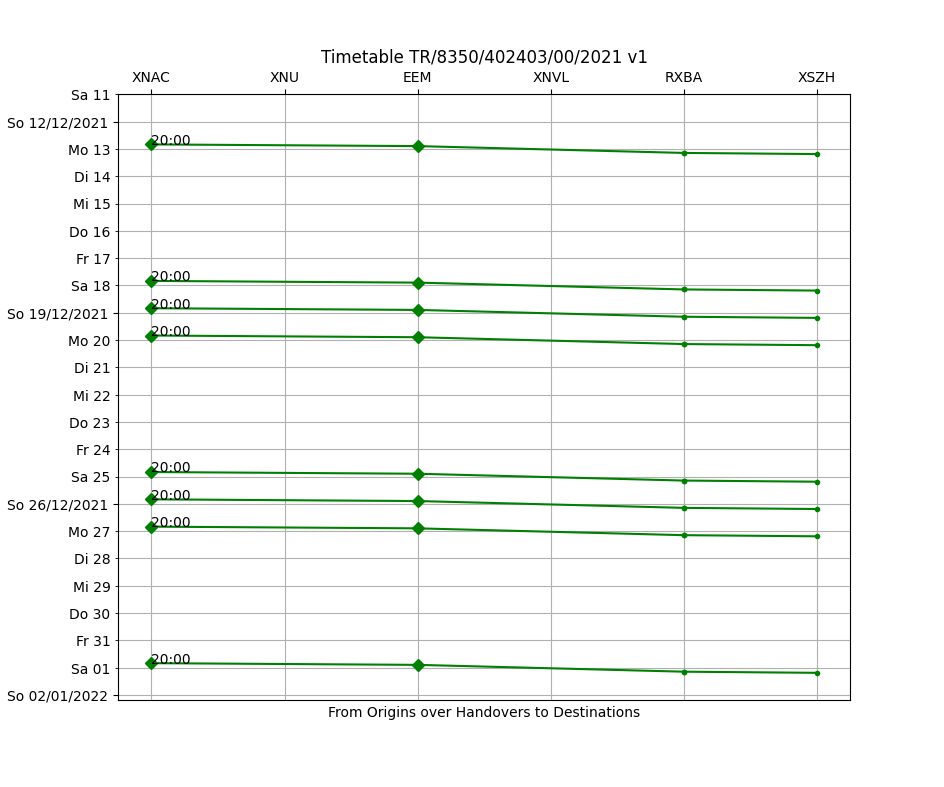
Route Sections¶
From which sections the train is composed?
section: RouteSection
for section in t.sections:
print(section.description(), "\n")
Out:
ID : 10.v1
Calender : 12/12 to 31/12 10000111000011100001
Start at: 20:00 in XNAC
Arrival at: 21:30 in EEM
Successors: [11]
ID : 11.v1
Calender : 12/12 to 31/12 10000111000011100001
Start at: 21:30 in EEM
Arrival at: 03:30 in RXBA
Successors: [12]
ID : 12.v1
Calender : 13/12 to 01/01 10000111000011100001
Start at: 03:30 in RXBA
Arrival at: 04:30 in XSZH
Successors: []
Section graph¶
The section graph is computed using the successor relation:
sg = t.section_graph()
plot_graph(sg)
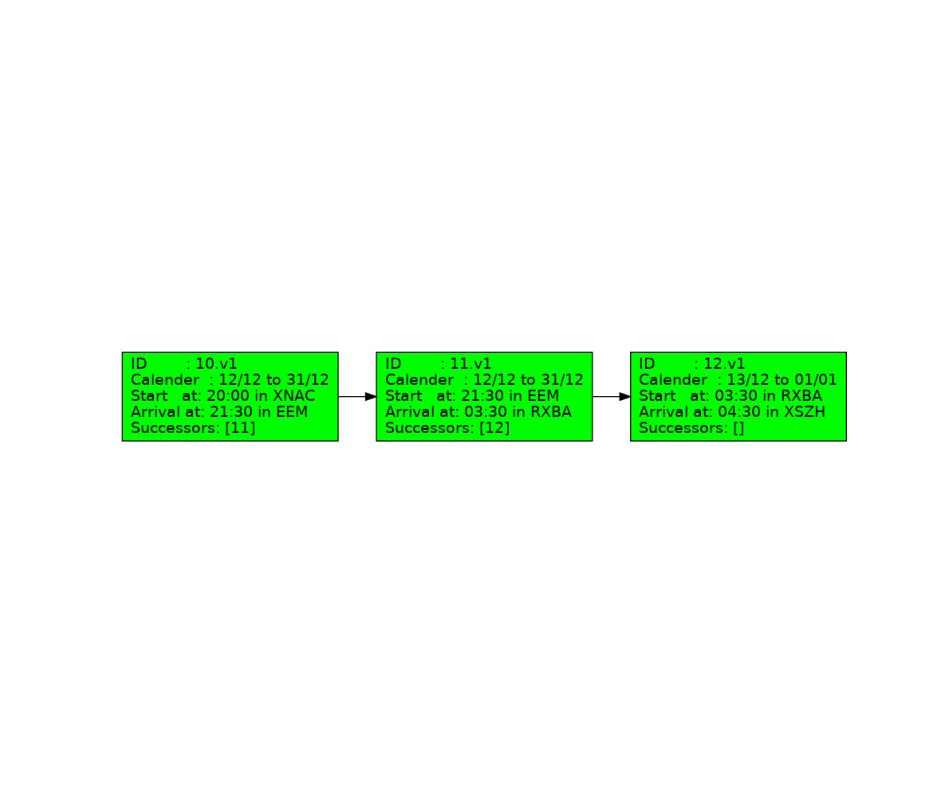
Routes¶
Print all possible routes. Routes are calculated from all possible paths in the section graph. Version 1 has only one route with three sections.
route: Route
for route in t.routes():
print(route.description(), "\n")
Out:
Route : XNAC-EEM-RXBA-XSZH
Key : 10-11-12
Calendar : 12/12 to 31/12 10000111000011100001
Start at: 20:00 in XNAC
Arrival at: 21:30 in EEM
Arrival at: 03:30 in RXBA
Arrival at: 04:30 in XSZH
Section runs¶
For each day of the calendar of a section a SectionRun is created. The section runs are the rows of RouteSection.to_dataframe. We only show the section run in december here.
for section in t.sections:
print(f"{section.section_id}: {section}")
print(section.to_dataframe(), "\n")
Out:
10: XNAC-EEM
ID XNAC EEM
2021-12-12 10 2021-12-12 20:00:00 2021-12-12 21:30:00
2021-12-17 10 2021-12-17 20:00:00 2021-12-17 21:30:00
2021-12-18 10 2021-12-18 20:00:00 2021-12-18 21:30:00
2021-12-19 10 2021-12-19 20:00:00 2021-12-19 21:30:00
2021-12-24 10 2021-12-24 20:00:00 2021-12-24 21:30:00
2021-12-25 10 2021-12-25 20:00:00 2021-12-25 21:30:00
2021-12-26 10 2021-12-26 20:00:00 2021-12-26 21:30:00
2021-12-31 10 2021-12-31 20:00:00 2021-12-31 21:30:00
11: EEM-RXBA
ID EEM RXBA
2021-12-12 11 2021-12-12 21:30:00 2021-12-13 03:30:00
2021-12-17 11 2021-12-17 21:30:00 2021-12-18 03:30:00
2021-12-18 11 2021-12-18 21:30:00 2021-12-19 03:30:00
2021-12-19 11 2021-12-19 21:30:00 2021-12-20 03:30:00
2021-12-24 11 2021-12-24 21:30:00 2021-12-25 03:30:00
2021-12-25 11 2021-12-25 21:30:00 2021-12-26 03:30:00
2021-12-26 11 2021-12-26 21:30:00 2021-12-27 03:30:00
2021-12-31 11 2021-12-31 21:30:00 2022-01-01 03:30:00
12: RXBA-XSZH
ID RXBA XSZH
2021-12-13 12 2021-12-13 03:30:00 2021-12-13 04:30:00
2021-12-18 12 2021-12-18 03:30:00 2021-12-18 04:30:00
2021-12-19 12 2021-12-19 03:30:00 2021-12-19 04:30:00
2021-12-20 12 2021-12-20 03:30:00 2021-12-20 04:30:00
2021-12-25 12 2021-12-25 03:30:00 2021-12-25 04:30:00
2021-12-26 12 2021-12-26 03:30:00 2021-12-26 04:30:00
2021-12-27 12 2021-12-27 03:30:00 2021-12-27 04:30:00
2022-01-01 12 2022-01-01 03:30:00 2022-01-01 04:30:00
TrainRuns¶
Each TrainRun defines a row in the timetable of the train above.
tr: TrainRun
for tr in t.train_run_iterator():
print(tr)
for sr in tr.sections_runs:
print(sr)
print("\n")
Out:
TR/8350/402403/10/2021/2021-12-12
10.v1:2021-12-12 20:00 OTR=0 XNAC-EEM 2021-12-12 21:30 OTR=0
11.v1:2021-12-12 21:30 OTR=0 EEM-RXBA 2021-12-13 03:30 OTR=1
12.v1:2021-12-13 03:30 OTR=1 RXBA-XSZH 2021-12-13 04:30 OTR=1
TR/8350/402403/10/2021/2021-12-17
10.v1:2021-12-17 20:00 OTR=0 XNAC-EEM 2021-12-17 21:30 OTR=0
11.v1:2021-12-17 21:30 OTR=0 EEM-RXBA 2021-12-18 03:30 OTR=1
12.v1:2021-12-18 03:30 OTR=1 RXBA-XSZH 2021-12-18 04:30 OTR=1
TR/8350/402403/10/2021/2021-12-18
10.v1:2021-12-18 20:00 OTR=0 XNAC-EEM 2021-12-18 21:30 OTR=0
11.v1:2021-12-18 21:30 OTR=0 EEM-RXBA 2021-12-19 03:30 OTR=1
12.v1:2021-12-19 03:30 OTR=1 RXBA-XSZH 2021-12-19 04:30 OTR=1
TR/8350/402403/10/2021/2021-12-19
10.v1:2021-12-19 20:00 OTR=0 XNAC-EEM 2021-12-19 21:30 OTR=0
11.v1:2021-12-19 21:30 OTR=0 EEM-RXBA 2021-12-20 03:30 OTR=1
12.v1:2021-12-20 03:30 OTR=1 RXBA-XSZH 2021-12-20 04:30 OTR=1
TR/8350/402403/10/2021/2021-12-24
10.v1:2021-12-24 20:00 OTR=0 XNAC-EEM 2021-12-24 21:30 OTR=0
11.v1:2021-12-24 21:30 OTR=0 EEM-RXBA 2021-12-25 03:30 OTR=1
12.v1:2021-12-25 03:30 OTR=1 RXBA-XSZH 2021-12-25 04:30 OTR=1
TR/8350/402403/10/2021/2021-12-25
10.v1:2021-12-25 20:00 OTR=0 XNAC-EEM 2021-12-25 21:30 OTR=0
11.v1:2021-12-25 21:30 OTR=0 EEM-RXBA 2021-12-26 03:30 OTR=1
12.v1:2021-12-26 03:30 OTR=1 RXBA-XSZH 2021-12-26 04:30 OTR=1
TR/8350/402403/10/2021/2021-12-26
10.v1:2021-12-26 20:00 OTR=0 XNAC-EEM 2021-12-26 21:30 OTR=0
11.v1:2021-12-26 21:30 OTR=0 EEM-RXBA 2021-12-27 03:30 OTR=1
12.v1:2021-12-27 03:30 OTR=1 RXBA-XSZH 2021-12-27 04:30 OTR=1
TR/8350/402403/10/2021/2021-12-31
10.v1:2021-12-31 20:00 OTR=0 XNAC-EEM 2021-12-31 21:30 OTR=0
11.v1:2021-12-31 21:30 OTR=0 EEM-RXBA 2022-01-01 03:30 OTR=30
12.v1:2022-01-01 03:30 OTR=30 RXBA-XSZH 2022-01-01 04:30 OTR=30
RoutingInformation as TrainInformation¶
An XML Dump of the routing information of version 1
print(dump_routing_info_as_xml(t))
Out:
<TrainInformation xmlns="http://taf-jsg.info/schemes" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://taf-jsg.info/schemes file:///../tests/data/xml/taf_cat_complete_sector.xsd" RouteInfoVersion="1">
<RouteSection SectionVersion="1" HasReferenceCalender="true">
<SectionID>
<ObjectType>RS</ObjectType>
<Company>8350</Company>
<Core>------402403</Core>
<Variant>10</Variant>
<TimetableYear>2021</TimetableYear>
</SectionID>
<PlannedJourneyLocation>
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10014</LocationPrimaryCode>
<PrimaryLocationName>XNAC</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>20:00:00</Time>
<Offset>0</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
<PlannedJourneyLocation>
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10015</LocationPrimaryCode>
<PrimaryLocationName>EEM</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>21:30:00</Time>
<Offset>0</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
<PlannedCalendar>
<BitmapDays>10000111000011100001</BitmapDays>
<ValidityPeriod>
<StartDateTime>2021-12-12T00:00:00</StartDateTime>
<EndDateTime>2021-12-31T00:00:00</EndDateTime>
</ValidityPeriod>
</PlannedCalendar>
<Successors>
<SectionID>
<ObjectType>RS</ObjectType>
<Company>8350</Company>
<Core>------402403</Core>
<Variant>11</Variant>
<TimetableYear>2021</TimetableYear>
</SectionID>
</Successors>
</RouteSection>
<RouteSection SectionVersion="1">
<SectionID>
<ObjectType>RS</ObjectType>
<Company>8350</Company>
<Core>------402403</Core>
<Variant>11</Variant>
<TimetableYear>2021</TimetableYear>
</SectionID>
<PlannedJourneyLocation>
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10015</LocationPrimaryCode>
<PrimaryLocationName>EEM</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>21:30:00</Time>
<Offset>0</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
<PlannedJourneyLocation>
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10016</LocationPrimaryCode>
<PrimaryLocationName>RXBA</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>03:30:00</Time>
<Offset>1</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
<PlannedCalendar>
<BitmapDays>10000111000011100001</BitmapDays>
<ValidityPeriod>
<StartDateTime>2021-12-12T00:00:00</StartDateTime>
<EndDateTime>2021-12-31T00:00:00</EndDateTime>
</ValidityPeriod>
</PlannedCalendar>
<Successors>
<SectionID>
<ObjectType>RS</ObjectType>
<Company>8350</Company>
<Core>------402403</Core>
<Variant>12</Variant>
<TimetableYear>2021</TimetableYear>
</SectionID>
</Successors>
</RouteSection>
<RouteSection SectionVersion="1">
<SectionID>
<ObjectType>RS</ObjectType>
<Company>8350</Company>
<Core>------402403</Core>
<Variant>12</Variant>
<TimetableYear>2021</TimetableYear>
</SectionID>
<PlannedJourneyLocation>
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10016</LocationPrimaryCode>
<PrimaryLocationName>RXBA</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>03:30:00</Time>
<Offset>0</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
<PlannedJourneyLocation>
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10017</LocationPrimaryCode>
<PrimaryLocationName>XSZH</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>04:30:00</Time>
<Offset>0</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
<PlannedCalendar>
<BitmapDays>10000111000011100001</BitmapDays>
<ValidityPeriod>
<StartDateTime>2021-12-13T00:00:00</StartDateTime>
<EndDateTime>2022-01-01T00:00:00</EndDateTime>
</ValidityPeriod>
</PlannedCalendar>
</RouteSection>
<Route key="10-11-12">
<PlannedCalendar>
<BitmapDays>10000111000011100001</BitmapDays>
<ValidityPeriod>
<StartDateTime>2021-12-12T00:00:00</StartDateTime>
<EndDateTime>2021-12-31T00:00:00</EndDateTime>
</ValidityPeriod>
</PlannedCalendar>
<PlannedJourneyLocation JourneyLocationTypeCode="01">
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10014</LocationPrimaryCode>
<PrimaryLocationName>XNAC</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>20:00:00</Time>
<Offset>0</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
<PlannedJourneyLocation JourneyLocationTypeCode="04">
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10015</LocationPrimaryCode>
<PrimaryLocationName>EEM</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>21:30:00</Time>
<Offset>0</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
<PlannedJourneyLocation JourneyLocationTypeCode="04">
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10016</LocationPrimaryCode>
<PrimaryLocationName>RXBA</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>03:30:00</Time>
<Offset>1</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
<PlannedJourneyLocation JourneyLocationTypeCode="03">
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10017</LocationPrimaryCode>
<PrimaryLocationName>XSZH</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>04:30:00</Time>
<Offset>0</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
</Route>
</TrainInformation>
Update schedule (version 2)¶
Version 2 of the train contains the update from april on.
_, t_spec_file = example('../tests/data', 'ac-zue-2')
print(t_spec_file.read_text())
t = make_train_from_yml(t_spec_file)
Out:
---
# Nighttrain from Amsterdam to Zürich
coreID: 402403
version: 2
sections:
# Route Amsterdam-Emm-Basel-Zürich
- departure_station: XNAC
arrival_station: EEM
id: 10
version: 2
departure_time: '20:00:00'
travel_time: '01:30:00'
calendar:
begin: &b-begin '2022-04-12'
end: &b-end '2022-05-12'
mask: 'Fri Sat'
color: green
succ:
- 11
- departure_station: EEM
arrival_station: RXBA # Basel Grenze
id: 11
version: 1
travel_time: '06:00:00'
succ:
- 12
- departure_station: RXBA
arrival_station: XSZH # Zürich HB
id: 12
version: 1
travel_time: '01:00:00'
# Route Utrecht-Venlo-Basel
- departure_station: XNU # Utrecht
arrival_station: XNVL # Venlo
id: 20
version: 1
departure_time: '20:30:00'
travel_time: '01:30:00'
calendar:
begin: *b-begin
end: *b-end
mask: 'Sun'
color: red
succ:
- 21
- departure_station: XNVL
arrival_station: RXBA
id: 21
version: 1
travel_time: '06:00:00'
succ:
- 12
Bildfahrplan¶
On sundays the trains only go to Basel from Utrecht over Venlo.
plot_train(t, all_stations=stations)
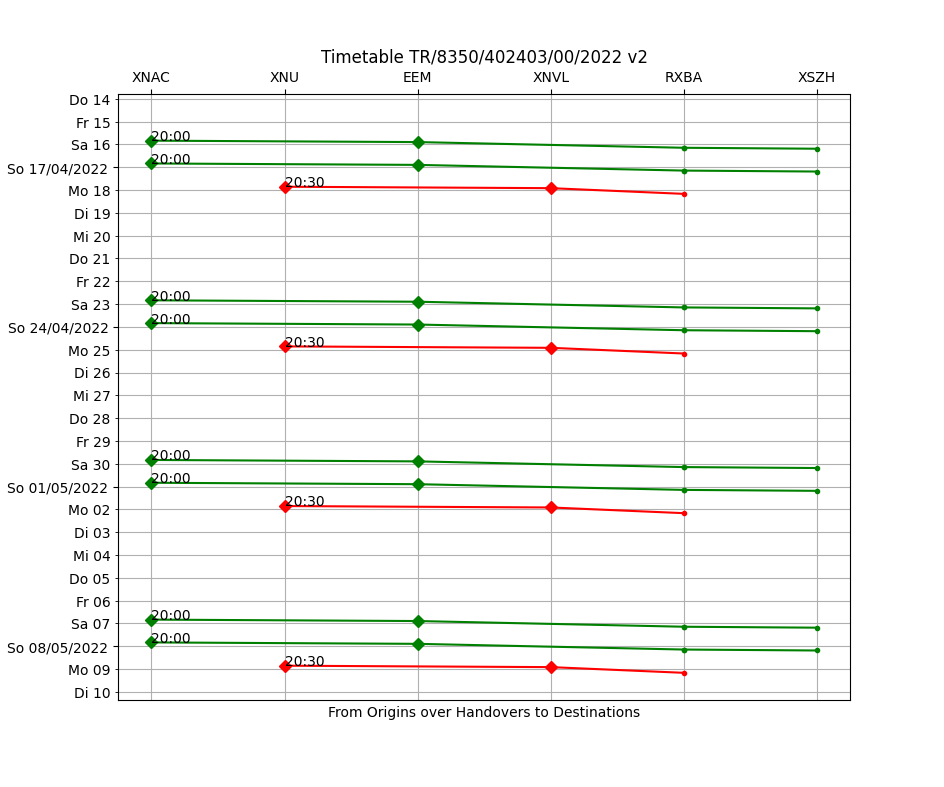
Route Sections¶
To realize the schedule we need two new section (with ID 20 and 40) All oteher section have a new version 2, because the calender had to shortened by sunday.
section: RouteSection
for section in t.sections:
print(section.description(), "\n")
Out:
ID : 10.v2
Calender : 15/04 to 07/05 11000001100000110000011
Start at: 20:00 in XNAC
Arrival at: 21:30 in EEM
Successors: [11]
ID : 11.v1
Calender : 15/04 to 07/05 11000001100000110000011
Start at: 21:30 in EEM
Arrival at: 03:30 in RXBA
Successors: [12]
ID : 12.v1
Calender : 16/04 to 08/05 11000001100000110000011
Start at: 03:30 in RXBA
Arrival at: 04:30 in XSZH
Successors: []
ID : 20.v1
Calender : 17/04 to 08/05 1000000100000010000001
Start at: 20:30 in XNU
Arrival at: 22:00 in XNVL
Successors: [21]
ID : 21.v1
Calender : 17/04 to 08/05 1000000100000010000001
Start at: 22:00 in XNVL
Arrival at: 04:00 in RXBA
Successors: [12]
Section graph¶
The section graph now has two new sections, which define the second route.
sg = t.section_graph()
plot_graph(sg)
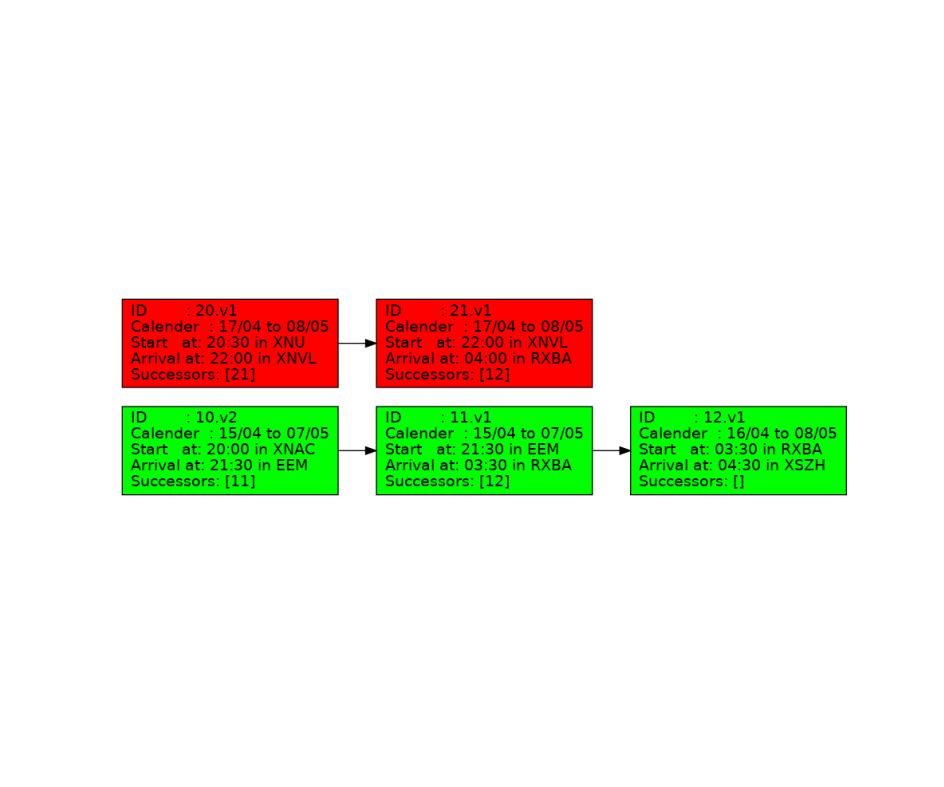
Routes¶
From april on we have one new route:
route: Route
for route in t.routes():
print(route.description(), "\n")
Out:
Route : XNAC-EEM-RXBA-XSZH
Key : 10-11-12
Calendar : 15/04 to 07/05 11000001100000110000011
Start at: 20:00 in XNAC
Arrival at: 21:30 in EEM
Arrival at: 03:30 in RXBA
Arrival at: 04:30 in XSZH
Route : XNU-XNVL-RXBA
Key : 20-21
Calendar : 17/04 to 08/05 1000000100000010000001
Start at: 20:30 in XNU
Arrival at: 22:00 in XNVL
Arrival at: 04:00 in RXBA
Section runs¶
for section in t.sections:
print(f"{section.section_id}: {section}")
print(section.to_dataframe(), "\n")
Out:
10: XNAC-EEM
ID XNAC EEM
2022-04-15 10 2022-04-15 20:00:00 2022-04-15 21:30:00
2022-04-16 10 2022-04-16 20:00:00 2022-04-16 21:30:00
2022-04-22 10 2022-04-22 20:00:00 2022-04-22 21:30:00
2022-04-23 10 2022-04-23 20:00:00 2022-04-23 21:30:00
2022-04-29 10 2022-04-29 20:00:00 2022-04-29 21:30:00
2022-04-30 10 2022-04-30 20:00:00 2022-04-30 21:30:00
2022-05-06 10 2022-05-06 20:00:00 2022-05-06 21:30:00
2022-05-07 10 2022-05-07 20:00:00 2022-05-07 21:30:00
11: EEM-RXBA
ID EEM RXBA
2022-04-15 11 2022-04-15 21:30:00 2022-04-16 03:30:00
2022-04-16 11 2022-04-16 21:30:00 2022-04-17 03:30:00
2022-04-22 11 2022-04-22 21:30:00 2022-04-23 03:30:00
2022-04-23 11 2022-04-23 21:30:00 2022-04-24 03:30:00
2022-04-29 11 2022-04-29 21:30:00 2022-04-30 03:30:00
2022-04-30 11 2022-04-30 21:30:00 2022-05-01 03:30:00
2022-05-06 11 2022-05-06 21:30:00 2022-05-07 03:30:00
2022-05-07 11 2022-05-07 21:30:00 2022-05-08 03:30:00
12: RXBA-XSZH
ID RXBA XSZH
2022-04-16 12 2022-04-16 03:30:00 2022-04-16 04:30:00
2022-04-17 12 2022-04-17 03:30:00 2022-04-17 04:30:00
2022-04-23 12 2022-04-23 03:30:00 2022-04-23 04:30:00
2022-04-24 12 2022-04-24 03:30:00 2022-04-24 04:30:00
2022-04-30 12 2022-04-30 03:30:00 2022-04-30 04:30:00
2022-05-01 12 2022-05-01 03:30:00 2022-05-01 04:30:00
2022-05-07 12 2022-05-07 03:30:00 2022-05-07 04:30:00
2022-05-08 12 2022-05-08 03:30:00 2022-05-08 04:30:00
20: XNU-XNVL
ID XNU XNVL
2022-04-17 20 2022-04-17 20:30:00 2022-04-17 22:00:00
2022-04-24 20 2022-04-24 20:30:00 2022-04-24 22:00:00
2022-05-01 20 2022-05-01 20:30:00 2022-05-01 22:00:00
2022-05-08 20 2022-05-08 20:30:00 2022-05-08 22:00:00
21: XNVL-RXBA
ID XNVL RXBA
2022-04-17 21 2022-04-17 22:00:00 2022-04-18 04:00:00
2022-04-24 21 2022-04-24 22:00:00 2022-04-25 04:00:00
2022-05-01 21 2022-05-01 22:00:00 2022-05-02 04:00:00
2022-05-08 21 2022-05-08 22:00:00 2022-05-09 04:00:00
TrainRuns¶
tr: TrainRun
for tr in t.train_run_iterator():
print(tr)
for sr in tr.sections_runs:
print(sr)
print("\n")
Out:
TR/8350/402403/10/2022/2022-04-15
10.v2:2022-04-15 20:00 OTR=0 XNAC-EEM 2022-04-15 21:30 OTR=0
11.v1:2022-04-15 21:30 OTR=0 EEM-RXBA 2022-04-16 03:30 OTR=1
12.v1:2022-04-16 03:30 OTR=1 RXBA-XSZH 2022-04-16 04:30 OTR=1
TR/8350/402403/10/2022/2022-04-16
10.v2:2022-04-16 20:00 OTR=0 XNAC-EEM 2022-04-16 21:30 OTR=0
11.v1:2022-04-16 21:30 OTR=0 EEM-RXBA 2022-04-17 03:30 OTR=1
12.v1:2022-04-17 03:30 OTR=1 RXBA-XSZH 2022-04-17 04:30 OTR=1
TR/8350/402403/10/2022/2022-04-22
10.v2:2022-04-22 20:00 OTR=0 XNAC-EEM 2022-04-22 21:30 OTR=0
11.v1:2022-04-22 21:30 OTR=0 EEM-RXBA 2022-04-23 03:30 OTR=1
12.v1:2022-04-23 03:30 OTR=1 RXBA-XSZH 2022-04-23 04:30 OTR=1
TR/8350/402403/10/2022/2022-04-23
10.v2:2022-04-23 20:00 OTR=0 XNAC-EEM 2022-04-23 21:30 OTR=0
11.v1:2022-04-23 21:30 OTR=0 EEM-RXBA 2022-04-24 03:30 OTR=1
12.v1:2022-04-24 03:30 OTR=1 RXBA-XSZH 2022-04-24 04:30 OTR=1
TR/8350/402403/10/2022/2022-04-29
10.v2:2022-04-29 20:00 OTR=0 XNAC-EEM 2022-04-29 21:30 OTR=0
11.v1:2022-04-29 21:30 OTR=0 EEM-RXBA 2022-04-30 03:30 OTR=1
12.v1:2022-04-30 03:30 OTR=1 RXBA-XSZH 2022-04-30 04:30 OTR=1
TR/8350/402403/10/2022/2022-04-30
10.v2:2022-04-30 20:00 OTR=0 XNAC-EEM 2022-04-30 21:30 OTR=0
11.v1:2022-04-30 21:30 OTR=0 EEM-RXBA 2022-05-01 03:30 OTR=29
12.v1:2022-05-01 03:30 OTR=29 RXBA-XSZH 2022-05-01 04:30 OTR=29
TR/8350/402403/10/2022/2022-05-06
10.v2:2022-05-06 20:00 OTR=0 XNAC-EEM 2022-05-06 21:30 OTR=0
11.v1:2022-05-06 21:30 OTR=0 EEM-RXBA 2022-05-07 03:30 OTR=1
12.v1:2022-05-07 03:30 OTR=1 RXBA-XSZH 2022-05-07 04:30 OTR=1
TR/8350/402403/10/2022/2022-05-07
10.v2:2022-05-07 20:00 OTR=0 XNAC-EEM 2022-05-07 21:30 OTR=0
11.v1:2022-05-07 21:30 OTR=0 EEM-RXBA 2022-05-08 03:30 OTR=1
12.v1:2022-05-08 03:30 OTR=1 RXBA-XSZH 2022-05-08 04:30 OTR=1
TR/8350/402403/20/2022/2022-04-17
20.v1:2022-04-17 20:30 OTR=0 XNU-XNVL 2022-04-17 22:00 OTR=0
21.v1:2022-04-17 22:00 OTR=0 XNVL-RXBA 2022-04-18 04:00 OTR=1
TR/8350/402403/20/2022/2022-04-24
20.v1:2022-04-24 20:30 OTR=0 XNU-XNVL 2022-04-24 22:00 OTR=0
21.v1:2022-04-24 22:00 OTR=0 XNVL-RXBA 2022-04-25 04:00 OTR=1
TR/8350/402403/20/2022/2022-05-01
20.v1:2022-05-01 20:30 OTR=0 XNU-XNVL 2022-05-01 22:00 OTR=0
21.v1:2022-05-01 22:00 OTR=0 XNVL-RXBA 2022-05-02 04:00 OTR=1
TR/8350/402403/20/2022/2022-05-08
20.v1:2022-05-08 20:30 OTR=0 XNU-XNVL 2022-05-08 22:00 OTR=0
21.v1:2022-05-08 22:00 OTR=0 XNVL-RXBA 2022-05-09 04:00 OTR=1
RoutingInformation as TrainInformation¶
An XML Dump of the routing information of version 1
print(dump_routing_info_as_xml(t))
Out:
<TrainInformation xmlns="http://taf-jsg.info/schemes" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://taf-jsg.info/schemes file:///../tests/data/xml/taf_cat_complete_sector.xsd" RouteInfoVersion="2">
<RouteSection SectionVersion="2" HasReferenceCalender="true">
<SectionID>
<ObjectType>RS</ObjectType>
<Company>8350</Company>
<Core>------402403</Core>
<Variant>10</Variant>
<TimetableYear>2022</TimetableYear>
</SectionID>
<PlannedJourneyLocation>
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10014</LocationPrimaryCode>
<PrimaryLocationName>XNAC</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>20:00:00</Time>
<Offset>0</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
<PlannedJourneyLocation>
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10015</LocationPrimaryCode>
<PrimaryLocationName>EEM</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>21:30:00</Time>
<Offset>0</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
<PlannedCalendar>
<BitmapDays>11000001100000110000011</BitmapDays>
<ValidityPeriod>
<StartDateTime>2022-04-15T00:00:00</StartDateTime>
<EndDateTime>2022-05-07T00:00:00</EndDateTime>
</ValidityPeriod>
</PlannedCalendar>
<Successors>
<SectionID>
<ObjectType>RS</ObjectType>
<Company>8350</Company>
<Core>------402403</Core>
<Variant>11</Variant>
<TimetableYear>2022</TimetableYear>
</SectionID>
</Successors>
</RouteSection>
<RouteSection SectionVersion="1">
<SectionID>
<ObjectType>RS</ObjectType>
<Company>8350</Company>
<Core>------402403</Core>
<Variant>11</Variant>
<TimetableYear>2022</TimetableYear>
</SectionID>
<PlannedJourneyLocation>
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10015</LocationPrimaryCode>
<PrimaryLocationName>EEM</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>21:30:00</Time>
<Offset>0</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
<PlannedJourneyLocation>
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10016</LocationPrimaryCode>
<PrimaryLocationName>RXBA</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>03:30:00</Time>
<Offset>1</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
<PlannedCalendar>
<BitmapDays>11000001100000110000011</BitmapDays>
<ValidityPeriod>
<StartDateTime>2022-04-15T00:00:00</StartDateTime>
<EndDateTime>2022-05-07T00:00:00</EndDateTime>
</ValidityPeriod>
</PlannedCalendar>
<Successors>
<SectionID>
<ObjectType>RS</ObjectType>
<Company>8350</Company>
<Core>------402403</Core>
<Variant>12</Variant>
<TimetableYear>2022</TimetableYear>
</SectionID>
</Successors>
</RouteSection>
<RouteSection SectionVersion="1">
<SectionID>
<ObjectType>RS</ObjectType>
<Company>8350</Company>
<Core>------402403</Core>
<Variant>12</Variant>
<TimetableYear>2022</TimetableYear>
</SectionID>
<PlannedJourneyLocation>
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10016</LocationPrimaryCode>
<PrimaryLocationName>RXBA</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>03:30:00</Time>
<Offset>0</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
<PlannedJourneyLocation>
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10017</LocationPrimaryCode>
<PrimaryLocationName>XSZH</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>04:30:00</Time>
<Offset>0</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
<PlannedCalendar>
<BitmapDays>11000001100000110000011</BitmapDays>
<ValidityPeriod>
<StartDateTime>2022-04-16T00:00:00</StartDateTime>
<EndDateTime>2022-05-08T00:00:00</EndDateTime>
</ValidityPeriod>
</PlannedCalendar>
</RouteSection>
<RouteSection SectionVersion="1" HasReferenceCalender="true">
<SectionID>
<ObjectType>RS</ObjectType>
<Company>8350</Company>
<Core>------402403</Core>
<Variant>20</Variant>
<TimetableYear>2022</TimetableYear>
</SectionID>
<PlannedJourneyLocation>
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10018</LocationPrimaryCode>
<PrimaryLocationName>XNU</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>20:30:00</Time>
<Offset>0</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
<PlannedJourneyLocation>
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10019</LocationPrimaryCode>
<PrimaryLocationName>XNVL</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>22:00:00</Time>
<Offset>0</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
<PlannedCalendar>
<BitmapDays>1000000100000010000001</BitmapDays>
<ValidityPeriod>
<StartDateTime>2022-04-17T00:00:00</StartDateTime>
<EndDateTime>2022-05-08T00:00:00</EndDateTime>
</ValidityPeriod>
</PlannedCalendar>
<Successors>
<SectionID>
<ObjectType>RS</ObjectType>
<Company>8350</Company>
<Core>------402403</Core>
<Variant>21</Variant>
<TimetableYear>2022</TimetableYear>
</SectionID>
</Successors>
</RouteSection>
<RouteSection SectionVersion="1">
<SectionID>
<ObjectType>RS</ObjectType>
<Company>8350</Company>
<Core>------402403</Core>
<Variant>21</Variant>
<TimetableYear>2022</TimetableYear>
</SectionID>
<PlannedJourneyLocation>
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10019</LocationPrimaryCode>
<PrimaryLocationName>XNVL</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>22:00:00</Time>
<Offset>0</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
<PlannedJourneyLocation>
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10016</LocationPrimaryCode>
<PrimaryLocationName>RXBA</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>04:00:00</Time>
<Offset>1</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
<PlannedCalendar>
<BitmapDays>1000000100000010000001</BitmapDays>
<ValidityPeriod>
<StartDateTime>2022-04-17T00:00:00</StartDateTime>
<EndDateTime>2022-05-08T00:00:00</EndDateTime>
</ValidityPeriod>
</PlannedCalendar>
<Successors>
<SectionID>
<ObjectType>RS</ObjectType>
<Company>8350</Company>
<Core>------402403</Core>
<Variant>12</Variant>
<TimetableYear>2022</TimetableYear>
</SectionID>
</Successors>
</RouteSection>
<Route key="10-11-12">
<PlannedCalendar>
<BitmapDays>11000001100000110000011</BitmapDays>
<ValidityPeriod>
<StartDateTime>2022-04-15T00:00:00</StartDateTime>
<EndDateTime>2022-05-07T00:00:00</EndDateTime>
</ValidityPeriod>
</PlannedCalendar>
<PlannedJourneyLocation JourneyLocationTypeCode="01">
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10014</LocationPrimaryCode>
<PrimaryLocationName>XNAC</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>20:00:00</Time>
<Offset>0</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
<PlannedJourneyLocation JourneyLocationTypeCode="04">
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10015</LocationPrimaryCode>
<PrimaryLocationName>EEM</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>21:30:00</Time>
<Offset>0</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
<PlannedJourneyLocation JourneyLocationTypeCode="04">
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10016</LocationPrimaryCode>
<PrimaryLocationName>RXBA</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>03:30:00</Time>
<Offset>1</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
<PlannedJourneyLocation JourneyLocationTypeCode="03">
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10017</LocationPrimaryCode>
<PrimaryLocationName>XSZH</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>04:30:00</Time>
<Offset>0</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
</Route>
<Route key="20-21">
<PlannedCalendar>
<BitmapDays>1000000100000010000001</BitmapDays>
<ValidityPeriod>
<StartDateTime>2022-04-17T00:00:00</StartDateTime>
<EndDateTime>2022-05-08T00:00:00</EndDateTime>
</ValidityPeriod>
</PlannedCalendar>
<PlannedJourneyLocation JourneyLocationTypeCode="01">
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10018</LocationPrimaryCode>
<PrimaryLocationName>XNU</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>20:30:00</Time>
<Offset>0</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
<PlannedJourneyLocation JourneyLocationTypeCode="04">
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10019</LocationPrimaryCode>
<PrimaryLocationName>XNVL</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>22:00:00</Time>
<Offset>0</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
<PlannedJourneyLocation JourneyLocationTypeCode="03">
<CountryCodeISO>DE</CountryCodeISO>
<LocationPrimaryCode>10016</LocationPrimaryCode>
<PrimaryLocationName>RXBA</PrimaryLocationName>
<TimingAtLocation>
<Timing>
<Time>04:00:00</Time>
<Offset>1</Offset>
</Timing>
</TimingAtLocation>
</PlannedJourneyLocation>
</Route>
</TrainInformation>
Total running time of the script: ( 0 minutes 1.581 seconds)